Using Html5-qrcode with only Camera or File options
01 Nov 2022 | Authored by Mohsina | Editted by MinhazHtml5-qrcode is a light-weight library to easily integrate QR code scanning functionality to any web application. You can find out more about the project and it’s implementation details on the project page on Github.
ScanApp is the end to end implementation of the library by the same author.
By default, the library allows two type of scanning options
- Camera based scan
- File based scan (Allows users to select any file on their device)
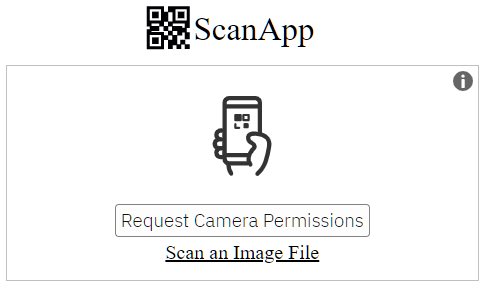
Earlier, there was no option to change this default configuration i.e. developers who wanted to use the library had to show both camera and file scan options even if their use-case was limited to one of them. There were feature request to open this up as a configuration.
The feature request
This feature request was raised seeking the support for controlling what all scan type should be rendered when using the library.
This seemed like an impactful item for me to start contributing to this project.
I added a configuration in Html5QrcodeScanner
to support this
I was able to add a new configuration option, such that the user can
- Either use only Camera based scan
- Or only File based scan
- Or use both of them, this happens to be the default option as well.
To configure the feature with Html5QrcodeScanner
, one need to simply set supportedScanTypes
options like this.
let html5QrcodeScanner = new Html5QrcodeScanner(
"reader",
{
fps: 10,
qrbox: qrboxFunction,
experimentalFeatures: {
useBarCodeDetectorIfSupported: true
},
rememberLastUsedCamera: true,
supportedScanTypes: [
Html5QrcodeScanType.SCAN_TYPE_FILE,
Html5QrcodeScanType.SCAN_TYPE_CAMERA
],
aspectRatio: 1.7777778
});
Note that
{
supportedScanTypes: [
Html5QrcodeScanType.SCAN_TYPE_FILE,
Html5QrcodeScanType.SCAN_TYPE_CAMERA
]
}
Is this the relevant configuration here. Here’s example of different scenarios.
[1] User only needs the camera based scanning.
In this case, set the supportedScanTypes
value like this.
supportedScanTypes: [ Html5QrcodeScanType.SCAN_TYPE_CAMERA ],
This will render the QR Scanner something like this
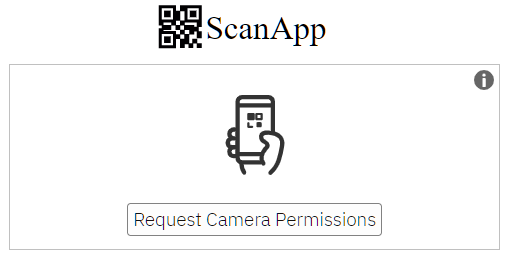
[2] User only needs the file based scanning.
Here set the supportedScanTypes
value like this.
{
supportedScanTypes: [Html5QrcodeScanType.SCAN_TYPE_FILE]
}
This will render the QR Scanner something like this
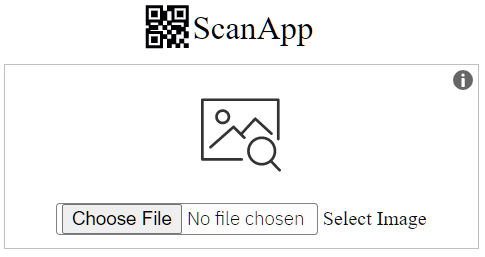
[3] User wants to support both camera and file based option
This is the default option! And unless you have specific need to disable one of the two, I recommend you to use this.
If we don’t set any value for supportedScanTypes
, the library will support
both scanning types by default.
Bonus: Set file based scanning as default option while supporting both
A bonus addition to the feature was that - the order of the config is also honoured by the library.
If you want to support both file and camera based scanning options but want to define file based option as the default you can just configure supportedScanTypes
like this
{
supportedScanTypes: [
Html5QrcodeScanType.SCAN_TYPE_FILE,
Html5QrcodeScanType.SCAN_TYPE_CAMERA
]
}
This will render the QR Scanner something like this
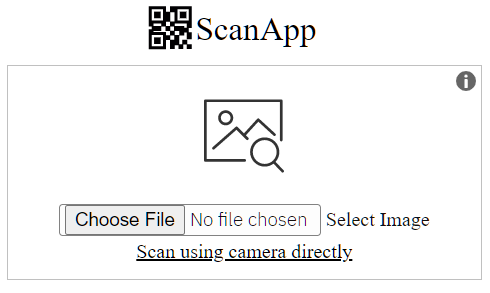
Otherwise, by default the library renders camera based option as the default one.
Similarly, you can define camera based option to be the default one
This happens to be the default scenario as well. So if you do nothing explicitly, library will behave in this fashion only.
{
supportedScanTypes: [
Html5QrcodeScanType.SCAN_TYPE_CAMERA,
Html5QrcodeScanType.SCAN_TYPE_FILE
]
}
If you pass arbitrary values, library will throw error!
I suppose this goes without saying!
If you provide values other than the supported ones - the library will throw error.
Summary
Developers can now modify the type of scanning method when using the Html5QrcodeScanner
library.
- Developers can choose to render only Camera support.
- Developers can choose to render only File selection support.
- Developers can choose to support both but select file or camera as the default option.
If you have any feedbacks, please share in comment section. For any bug report or feature request please report it to the html5-qrcode library directly.